Axios is a popular JavaScript library used for making HTTP requests. It is widely used in web development to fetch data from APIs and update the user interface accordingly. However, testing these requests can be difficult and time-consuming. This is where the Axios Mock Adapter comes in, providing an easy way to mock HTTP requests and responses.
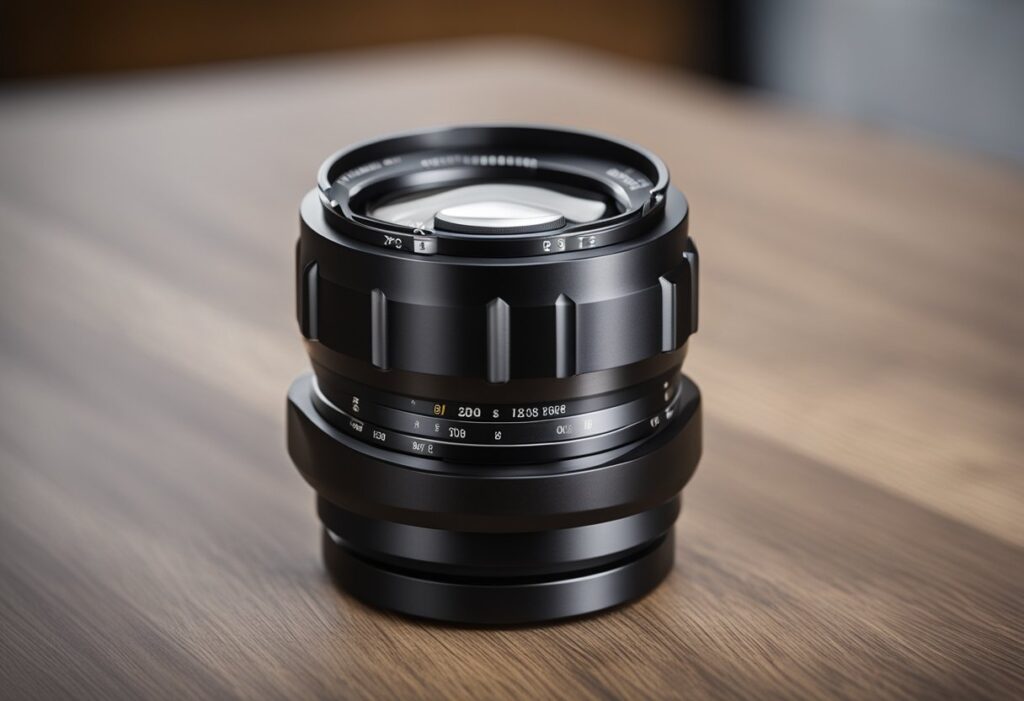
The Axios Mock Adapter is a utility that intercepts Axios requests and returns mock responses. This allows developers to test their code without making actual HTTP requests to a remote server. The mock adapter can be configured to return different responses based on the request method, URL, headers, and data. This makes it a powerful tool for testing different scenarios and edge cases.
Using the Axios Mock Adapter can save developers a lot of time and effort when testing their code. Instead of setting up a server or relying on a third-party API, developers can create mock responses that simulate different scenarios. This allows them to test their code in isolation and catch errors before they make it to production. Overall, the Axios Mock Adapter is a valuable tool for any developer working with Axios and HTTP requests.
Table of Contents
Understanding Axios Mock Adapter
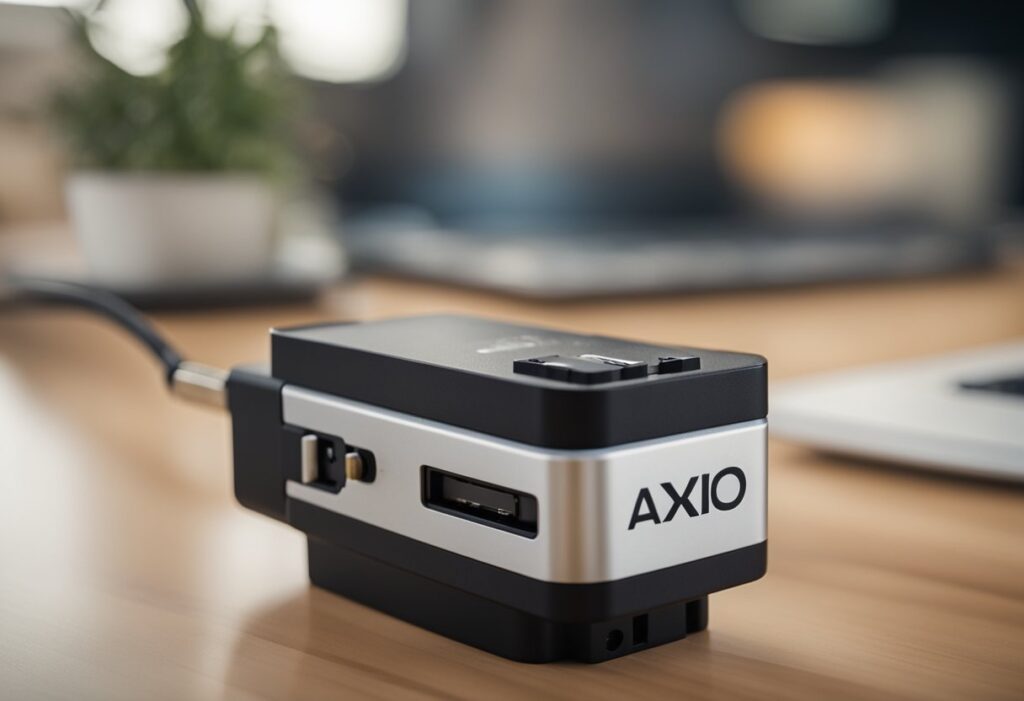
Axios Mock Adapter is a powerful tool for testing HTTP requests in JavaScript applications. With this tool, we can simulate responses from a server without actually making a request to the server. This allows us to test our code in isolation and without the need for a real server.
To use Axios Mock Adapter, we first need to create an instance of it. We can then use this instance to intercept HTTP requests and provide mock responses. Here’s an example:
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
const mock = new MockAdapter(axios);
mock.onGet('/users').reply(200, {
users: [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
],
});
axios.get('/users').then(response => {
console.log(response.data); // { users: [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' } ] }
});
In this example, we create a new instance of Axios Mock Adapter and intercept a GET request to /users
. We then provide a mock response with a status code of 200 and an object containing an array of users. When we make a request to /users
using axios.get
, we receive the mock response instead of making a real request to the server.
Axios Mock Adapter also allows us to simulate errors and delays in our responses. We can do this by using the replyWithError
and replyWithDelay
methods. Here’s an example:
mock.onGet('/users').replyWithError('Error');
axios.get('/users').catch(error => {
console.log(error.message); // 'Error'
});
mock.onGet('/users').replyWithDelay(1000, [200, { users: [] }]);
axios.get('/users').then(response => {
console.log(response.data); // { users: [] }
});
In this example, we first intercept a GET request to /users
and provide a mock response with an error. When we make a request to /users
using axios.get
, we catch the error and log the error message.
We then intercept another GET request to /users
and provide a mock response with a delay of 1 second and an empty array of users. When we make a request to /users
using axios.get
, we receive the mock response after a delay of 1 second.
Overall, Axios Mock Adapter is a powerful tool for testing HTTP requests in JavaScript applications. With this tool, we can simulate responses from a server without actually making a request to the server. This allows us to test our code in isolation and without the need for a real server.
Installation and Setup
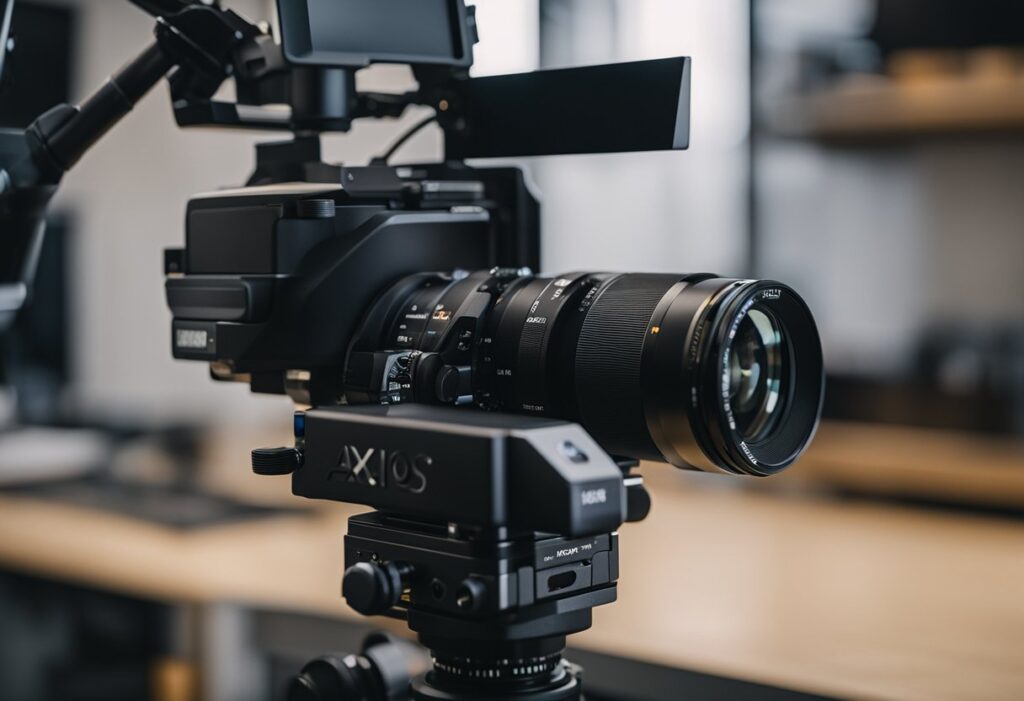
Installation
To use the Axios mock adapter, we first need to install it. We can do this by running the following command in our terminal:
npm install axios-mock-adapter --save-dev
This command will install the Axios mock adapter as a development dependency in our project.
Setup
Once we have installed the Axios mock adapter, we can start using it in our project. To set it up, we need to create an instance of the Axios mock adapter and pass it to our Axios instance.
Here is an example of how to set up the Axios mock adapter:
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
const mock = new MockAdapter(axios);
// Mock any GET request to /users
mock.onGet('/users').reply(200, {
users: [
{ id: 1, name: 'John Smith' },
{ id: 2, name: 'Jane Doe' },
],
});
In this example, we create a new instance of the Axios mock adapter and pass it to our Axios instance. We then mock any GET request to /users
and return a response with an array of users.
Now, when we make a GET request to /users
using Axios, the mock adapter will intercept the request and return the mocked response instead of making a real request to the server.
That’s it! We have successfully installed and set up the Axios mock adapter in our project.
Basic Usage
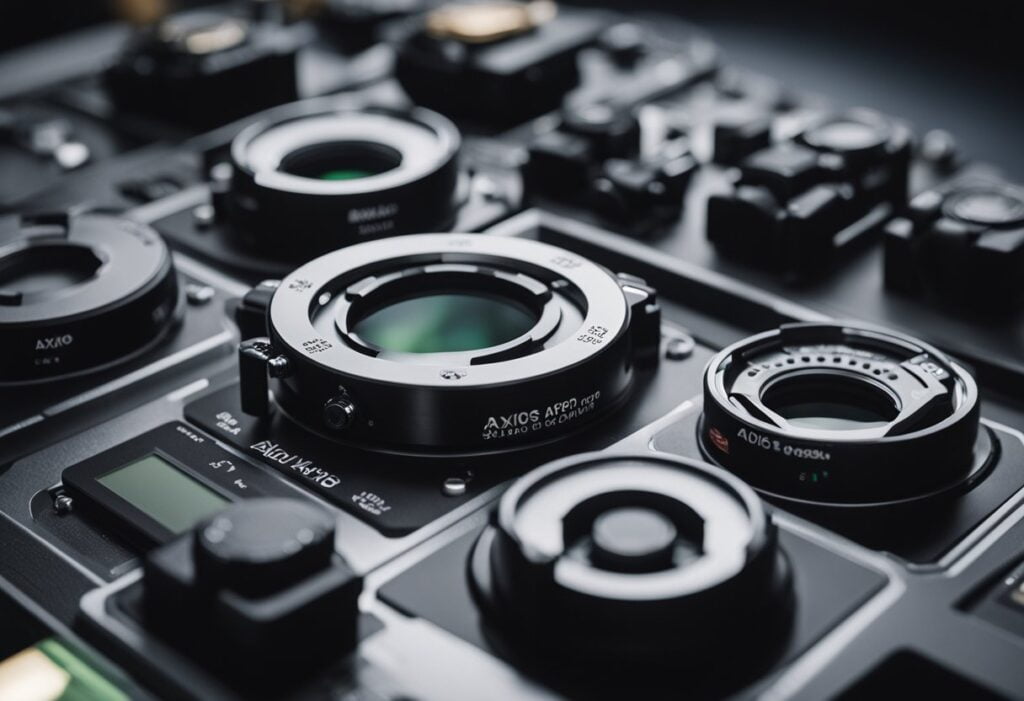
Creating an Instance
To use the Axios Mock Adapter, we first need to create an instance of it. We can do this by importing the axios
and axios-mock-adapter
libraries and then creating a new instance of the MockAdapter
class:
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
const mock = new MockAdapter(axios);
Once we have created the instance, we can use it to mock requests.
Mocking Requests
To mock a request, we can use the onGet
, onPost
, onPut
, onDelete
, and onAny
methods of the MockAdapter
instance. For example, to mock a GET request to the /users
endpoint, we can use the following code:
mock.onGet('/users').reply(200, {
users: [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
],
});
This will intercept any GET requests to the /users
endpoint and respond with a status code of 200 and a JSON object containing an array of two users.
We can also use regular expressions to match URLs. For example, to mock any GET request to an endpoint that starts with /users/
, we can use the following code:
mock.onGet(/^\/users\//).reply(200, {
message: 'This is a mock response for any user endpoint',
});
This will intercept any GET requests to any endpoint that starts with /users/
and respond with a status code of 200 and a JSON object containing a message.
In summary, the Axios Mock Adapter is a powerful tool for mocking HTTP requests in unit tests. We can create an instance of it and use it to intercept requests and respond with mock data.
Advanced Features
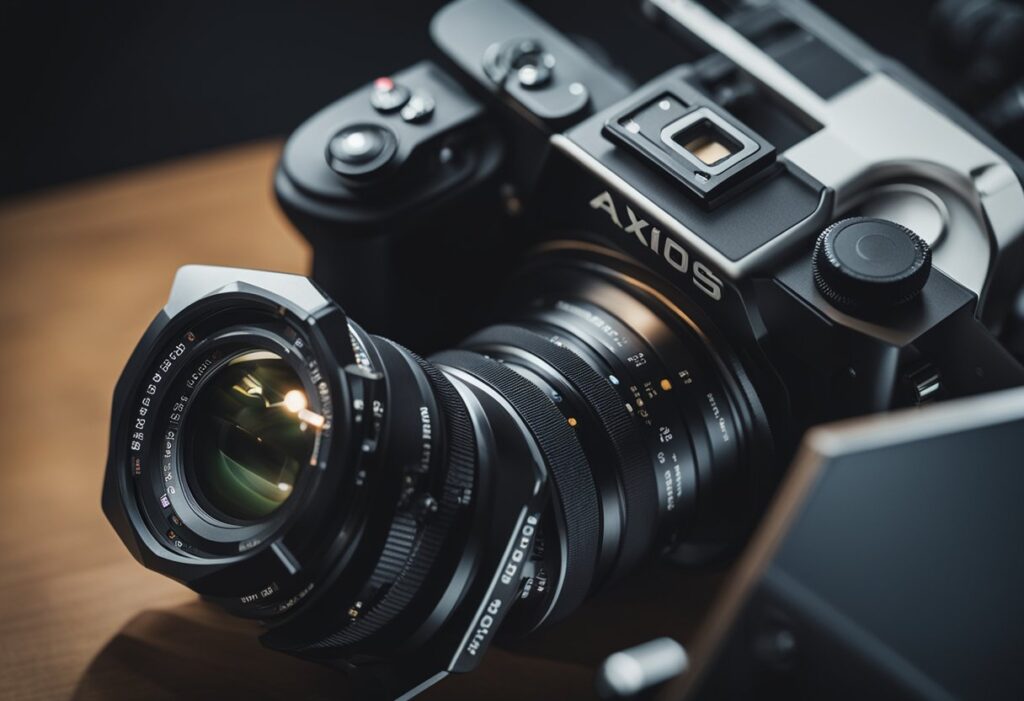
Simulating Network Errors
With Axios Mock Adapter, we can simulate network errors to test how our application handles them. We can do this by calling the networkError()
method on the mock adapter instance. This will return a rejected Promise with a network error object. We can also pass in a custom error message as a parameter to the networkError()
method.
mockAdapter.networkError();
Setting Default Responses
We can set default responses for all requests that match a certain URL or method. This is useful when we want to mock a specific API endpoint and don’t want to define a response for every single request that hits that endpoint. We can do this by calling the onAny()
method on the mock adapter instance and passing in the URL or method we want to match, along with the default response.
mockAdapter.onAny('/api/users').reply(200, {
users: [{ id: 1, name: 'John Doe' }]
});
Chaining Requests
We can chain requests together using Axios Mock Adapter to simulate a series of API calls. This is useful when we want to test how our application handles multiple requests that depend on each other. We can do this by calling the onGet()
or onPost()
method on the mock adapter instance and passing in a response that includes a URL that matches the next request.
mockAdapter.onGet('/api/users').reply(200, {
users: [{ id: 1, name: 'John Doe' }]
});
mockAdapter.onGet('/api/users/1').reply(200, {
id: 1,
name: 'John Doe',
email: 'john.doe@example.com'
});
In the example above, the first request to /api/users
will return a response with a user object that includes an id
property of 1
. The second request to /api/users/1
will match the id
property in the response from the first request and return a response with the full user object.
Testing with Axios Mock Adapter
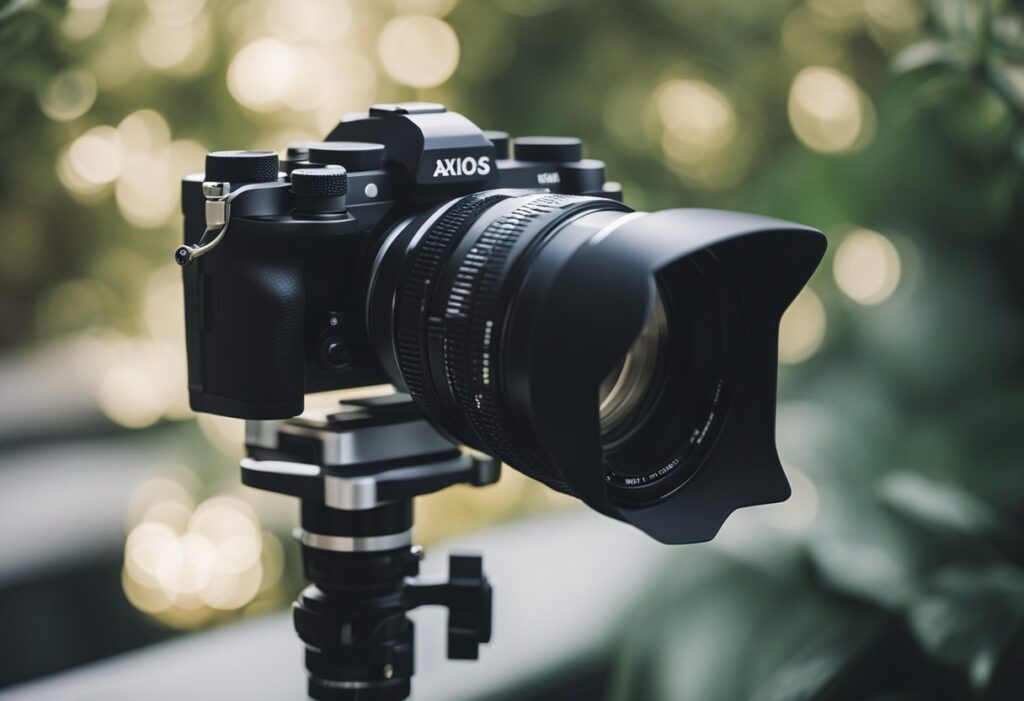
When it comes to testing our applications, we need to be able to mock API requests and responses to ensure that our code works as expected. This is where Axios Mock Adapter comes into play. Axios Mock Adapter is a library that allows us to easily mock HTTP requests made with Axios.
Unit Testing
Unit testing is a type of testing that focuses on testing individual units of code in isolation. When it comes to testing with Axios Mock Adapter, unit testing is the perfect way to test individual functions that make HTTP requests.
To use Axios Mock Adapter in unit tests, we need to create a new instance of the adapter and use it to mock our requests. We can then test our functions to ensure that they are making the correct requests and handling the responses correctly.
Integration Testing
Integration testing is a type of testing that focuses on testing how different parts of our application work together. When it comes to testing with Axios Mock Adapter, integration testing is the perfect way to test how our application interacts with APIs.
To use Axios Mock Adapter in integration tests, we need to create a new instance of the adapter and use it to mock our requests. We can then test our application to ensure that it is making the correct requests and handling the responses correctly.
Overall, Axios Mock Adapter is a powerful tool that can help us test our applications with ease. By using it in our unit and integration tests, we can ensure that our code is working as expected and that our application is interacting with APIs correctly.
Best Practices
Maintaining Code Quality
When using Axios Mock Adapter, it’s important to maintain code quality to ensure that the code is readable, maintainable, and scalable. Here are some best practices to follow:
- Write clear and concise code: Write code that is easy to understand and maintain. Use meaningful variable and function names, and avoid using unnecessary comments.
- Follow a consistent coding style: Follow a consistent coding style across your project to make it easier for other developers to read and understand your code. Use tools like ESLint to enforce coding standards.
- Write unit tests: Write unit tests to ensure that your code works as expected. Use tools like Jest to write and run tests.
Performance Optimization
Axios Mock Adapter can be used to simulate API responses, but it’s important to ensure that the performance of your application is not affected. Here are some best practices to follow:
- Use realistic response times: Use realistic response times when simulating API responses. This will help ensure that your application behaves as expected in production.
- Limit the number of requests: Limit the number of requests that are made to the mock API. This will help ensure that the performance of your application is not affected.
- Use caching: Use caching to improve the performance of your application. Use tools like Redis to cache responses and reduce the number of requests made to the mock API.
Following these best practices will help ensure that your application is scalable, maintainable, and performs well when using Axios Mock Adapter.
Common Pitfalls and How to Avoid Them
When working with the Axios mock adapter, there are a few common pitfalls that developers may encounter. Here are some tips on how to avoid them:
1. Forgetting to Reset the Adapter
One of the most common mistakes when using the Axios mock adapter is forgetting to reset it after each test. This can lead to unexpected behavior in subsequent tests, as the adapter may still be using data from a previous test.
To avoid this, we recommend resetting the adapter in the beforeEach
method of your test suite. This ensures that the adapter is always in a clean state before each test is run.
2. Not Mocking All Requests
Another common pitfall is not mocking all requests made by your application. If you forget to mock a request, your tests may fail or behave unexpectedly.
To avoid this, we recommend creating a comprehensive list of all requests made by your application and mocking each one in your test suite. This ensures that all requests are properly handled and your tests are reliable.
3. Using Incorrect Mock Data
When mocking requests, it’s important to use the correct data to ensure that your tests accurately reflect the behavior of your application. Using incorrect data can lead to false positives or false negatives in your test results.
To avoid this, we recommend carefully reviewing the data used in your mock requests and ensuring that it accurately reflects the data used by your application.
4. Failing to Handle Errors
Finally, it’s important to properly handle errors when working with the Axios mock adapter. If errors are not handled correctly, your tests may fail or behave unexpectedly.
To avoid this, we recommend testing both successful and unsuccessful requests in your test suite. This ensures that your application handles errors correctly and your tests are reliable.
By following these tips, you can avoid common pitfalls when working with the Axios mock adapter and ensure that your tests are reliable and accurate.
Frequently Asked Questions
How to use Axios mock adapter?
Using Axios mock adapter is simple. First, you need to import the axios-mock-adapter
library and create a new instance of it. Then, you can use the onGet
, onPost
, onPut
, onDelete
, and onAny
methods to define mock responses for specific HTTP methods or any HTTP method.
What is the difference between reset and restore in Axios mock adapter?
The reset
method clears all the mock handlers and resets the adapter to its initial state. The restore
method restores the original axios
instance and clears all the mock handlers.
What are some common issues with Axios-mock-adapter and how to fix them?
One common issue is forgetting to call the axios-mock-adapter
instance’s reset
method after each test in order to clear the mock handlers. Another issue is not defining a mock response for a specific HTTP method. To fix these issues, make sure to call the reset
method after each test and define mock responses for all the HTTP methods you need to mock.
How to use Axios-mock-adapter with Jest?
To use Axios-mock-adapter with Jest, you can create a new instance of the adapter in a beforeEach
hook and call its reset
method after each test. You can also define your mock responses in the same hook.
What is the purpose of Axios mock adapter?
The purpose of Axios mock adapter is to mock HTTP requests and responses in order to test the behavior of code that uses the Axios library without actually making real HTTP requests.
How to mock Axios interceptors?
To mock Axios interceptors, you can define mock responses for requests that match the interceptor’s criteria. For example, if you have an interceptor that adds an authorization header to all requests, you can define a mock response for a request that includes that header.